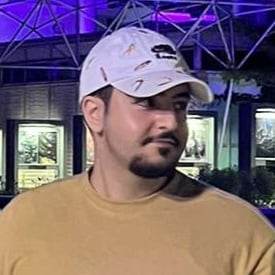
The Basics: JavaScript Strings
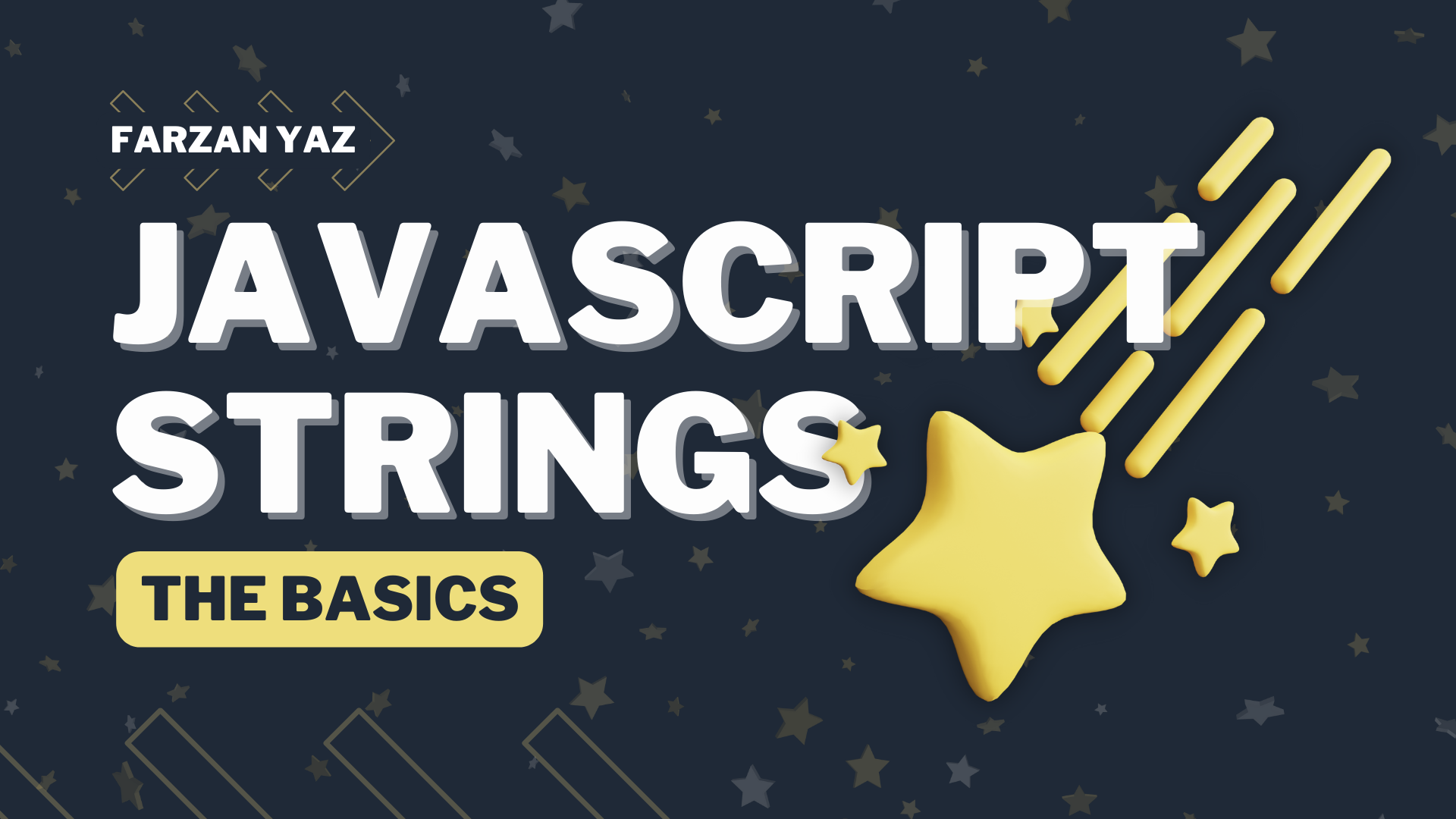
In this post, we'll be covering the basics of JavaScript Strings and a variety of the methods available to us. We'll also explore the template literal - a relatively newer string format.
Introduction
A string is a sequence of one or more characters that may consist of letters, numbers, or symbols. In JavaScript, strings are primitive data types and immutable - meaning they are unchanging. Like most programming languages, a JavaScript string is structured as zero or more characters surrounded by quotes. Strings using single quotes and double quote are effectively the same - just make sure to keep your quote usage consistent throughout project files.
"This is an example of a JavaScript string!"
'This is also an example of a JavaScript string!'
View String Output
The easiest way to view the output of a string is to print it to your console using console.log().
console.log("This is a string!");
This is a string!
Note that the string written in the source code is called a string literal, while what we see in the output is called a string value.
Storing a String
In JavaScript, you can assign the value of a string to a named variable. Variables are containers that store a value, using keywords const, let, and less commonly var.
const myString = "Wow, a string assigned to a named variable!";
We can then reference this variable and print it to the console similarly to before.
console.log(myString);
Wow, a string assigned to a named variable!
String Concatenation
String concatenation refers to the joining of two or more strings to create a new string. In JavaScript, the concatenation operator is simply the + symbol. Let's take a look at an example - we'll assume that the results are console logged from now on.
"Hello" + "world!"
Helloworld!
Oops - that doesn't look quite right. Let's add an empty space at the end of the first term to fix this.
"Hello " + "world!"
Hello world!
We can also concatenate strings and variables containing string values.
const movie = "Iron Man 1";
const actor = "Robert Downey Jr.";
const favMovie = "My favorite " + actor + " movie is " + movie + ".";
My favorite Robert Downey Jr. movie is Iron Man 1.
Template Literal
Other than single and double quotes, the third and newest way to create a string is called a template literal. Template literals use the backtick (`) and work the same as regular strings with a notable bonus. Instead of using the concatenation operator as we do with regular strings, we can use the ${} syntax to insert a variable into the string.
const movie = "Iron Man 1";
const actor = "Robert Downey Jr.";
const favMovie = `My favorite ${actor} movie is ${movie}.`
My favorite Robert Downey Jr. movie is Iron Man 1.
Personally, I tend to opt for template literals over the concatenation operator as they provide a more convenient way of combining text and variables.
Quotes within Strings
If we want to use quotes within our string - such as an apostrophe or to denote dialogue - we run into runtime errors.
const brokenString = 'I'm a (heart)broken string...';
console.log(brokenString);
Unexpected token (1:24)
There are multiple ways around this. Firstly, we can alternate single and double quotes. For example, if we want to use single quotes within our string, we create our string using double quotes. And vice-versa.
"I'm a valid string with an apostrophe!"
'She told me, "the world is yours".'
This works great - however, if we want to stay consistent with our quotes, we need to use the backslash escape character (\) to prevent JavaScript from interpreting a quote as the end of the string.
'I\'m still a valid string with an apostrophe!'
"She told me, \"the world is yours\"."
Lastly, you can use single or double quotes within your string if it's created as a template literal using backticks.
`I'm a valid string using an apostrophe and "quotes" in a template literal.`
Long Strings and Newlines
To insert a newline character in your string, simply use \n.
const twoLines = "This is a string that\nspans across two lines.";
This is a string
that spans across two lines.
For longer, multi-line strings, you may want to opt for a template literal string.
const threeLines = `This is a string
that spans across
three lines.`;
This is a string
that spans across
three lines.
String Methods
Now that we have the basics out of the way, let's quickly take a look at the arsenal of string methods available to us through some examples. I've prepared the following cheat sheet to make it easier to find what you're looking for!
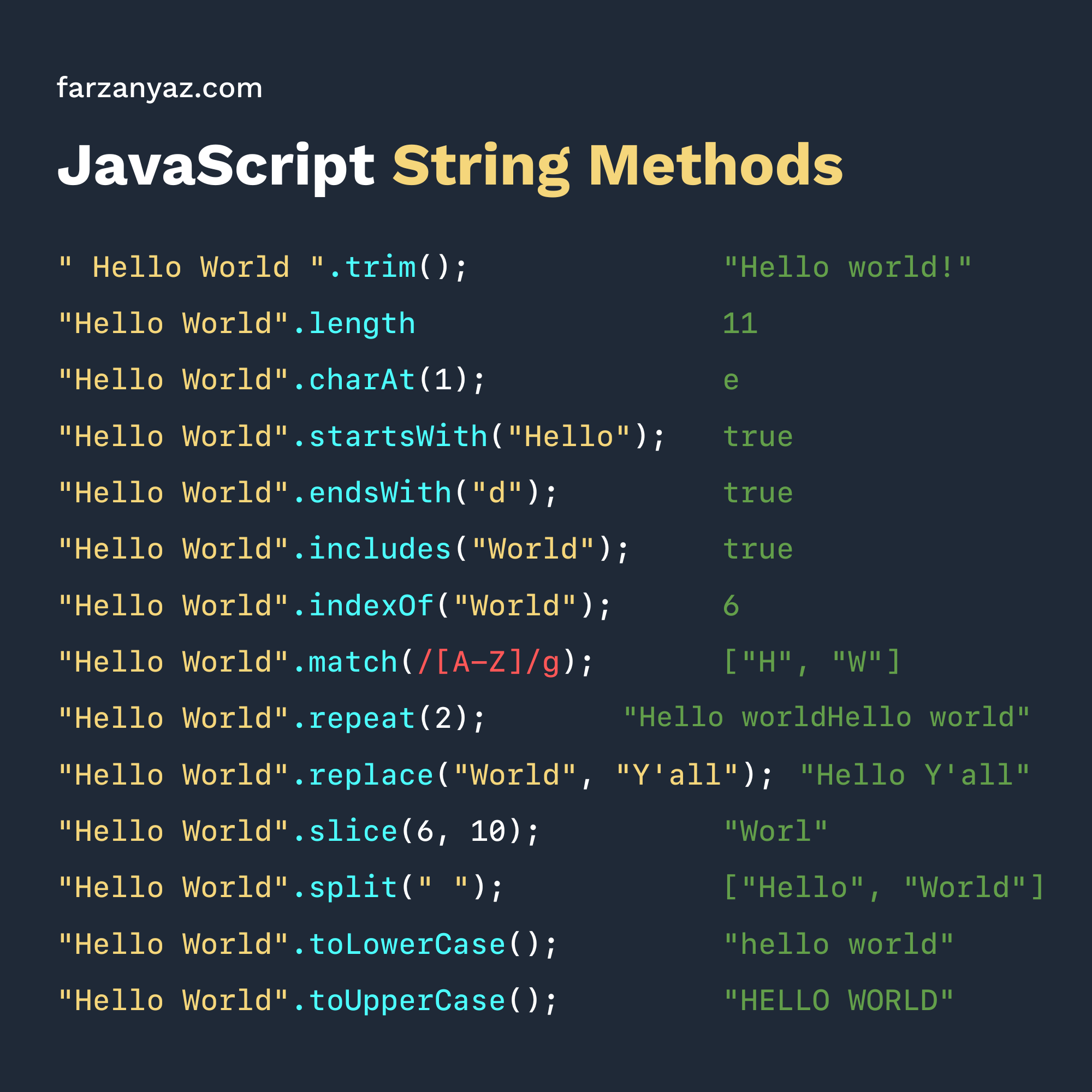
Conclusion
That's it for this introduction to JavaScript strings! We covered what a string is, outputting a string to the console, concatenating strings and variables, the template literal, how to use quotes within strings, multi-line strings, and finally a variety of string methods. I hope this post has helped you become more comfortable dealing with strings or has revealed a trick that you hadn't thought of before. Feel free to save the string methods cheat sheet for later review!